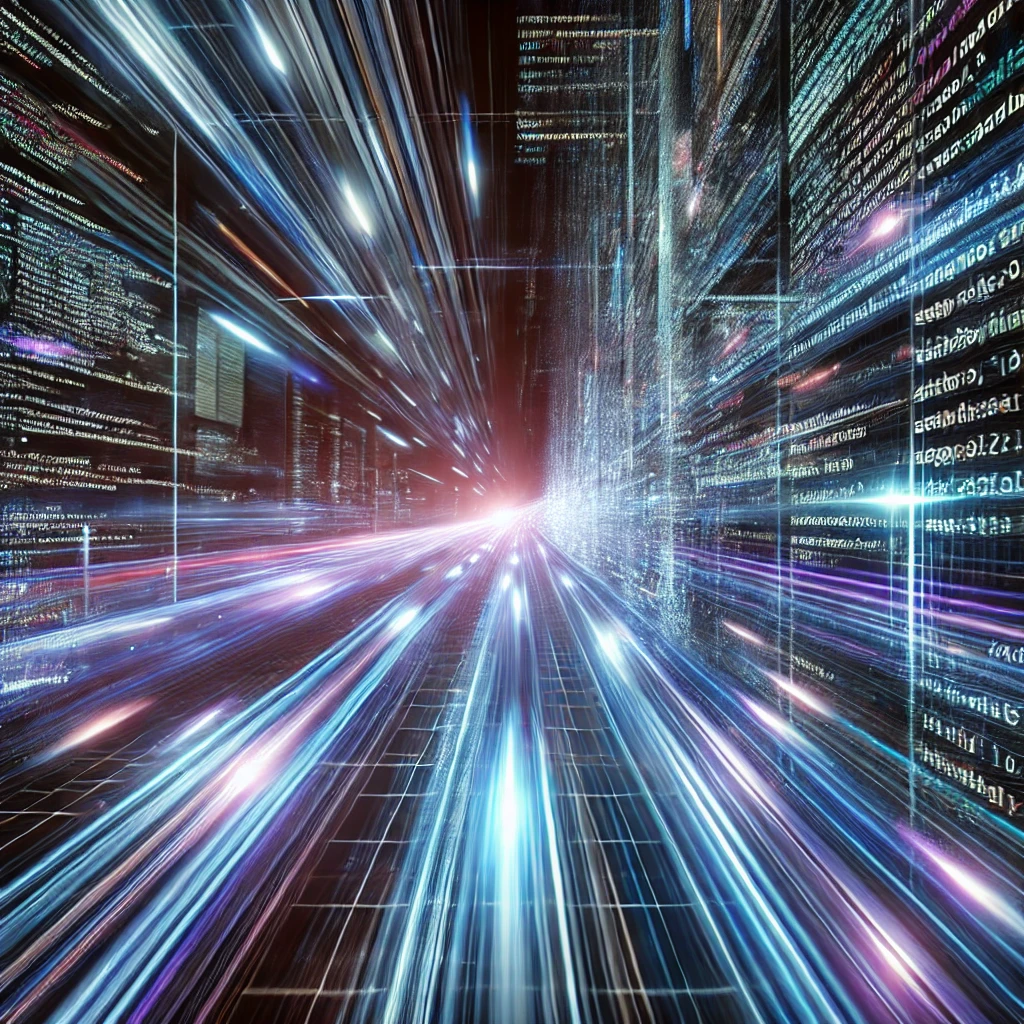
When developing SOLIDWORKS macros and add-ins, performance optimization is crucial. Slow execution times can frustrate users and reduce productivity, especially when automating complex operations. Fortunately, the SOLIDWORKS API provides several methods to enhance speed and efficiency.
Here are some of the SOLIDWORKS API calls that improve performance, with a focus on minimizing unnecessary computations, UI updates, and background processes.
1. What is CommandInProgress
?
CommandInProgress
is a property that tells SOLIDWORKS that an API-driven command is actively running. This prevents unnecessary UI updates and can significantly improve macro execution speed.
Use it:
- When executing long-running API tasks.
- When making multiple changes to a model in one go.
- When performing bulk operations like inserting components, suppressing features, or modifying multiple sketches.
Do not forget to set CommandInProgress to false when done. If not, some elements of the SOLIDWORKS UI will be disabled.
2. DoEvents
This is a VBA and VB.NET call. It ensures the UI remains responsive during long operations. If called too frequently, it can significantly slow down performance. Call it within a loop to allow the UI to update, but avoid excessive use. If you are looking for maximum performance, do not use DoEvents.
Example:
1 2 3 4 5 |
For i = 1 To 10000 ' Perform some operation DoEvents ' Allows UI updates, but slows performance if overused Next i |
3. AddToDB
One of the benefits of this API call is adding sketch entities directly to the database so that you can avoid grid and entity snapping. For example, if you create a sketch line whose endpoint is near another entity or grid point, the new sketch line endpoint snaps to the other entity or grid point. Setting this property to true avoids this behavior during sketch entity creation.
Example:
1 2 3 4 5 6 7 |
Dim swSketchManager as SketchManager swSketchManager.AddToDB = True ' Enable AddToDB for performance boost ' Create sketch entities here... swSketchManager.AddToDB = False ' Restore default behavior |
4. DisplayWhenAdded
This API call prevents SOLIDWORKS from drawing new sketch objects until explicitly requested. It must be manually disabled afterward, or the user will need to rebuild manually.
Example:
1 2 3 4 5 6 7 8 |
Dim swSketchMgr As SketchManager Set swSketchMgr = swModel.SketchManager swSketchMgr.DisplayWhenAdded = False ' Disable real-time drawing ' Create sketch entities... swSketchMgr.DisplayWhenAdded = True ' Restore default behavior |
5. EnableFeatureTree
This API call stops the feature tree from updating unnecessarily, improving execution speed.
Example:
1 2 3 4 5 6 7 8 |
Dim swFeatureMgr As FeatureManager Set swFeatureMgr = swModel.FeatureManager swFeatureMgr.EnableFeatureTree = False ' Disable feature tree updates ' Perform operations... swFeatureMgr.EnableFeatureTree = True ' Enable updates again |
6. EnableConfigurationTree
This property prevents configuration updates during a macro execution.
Example:
1 2 3 4 5 6 7 8 |
Dim swConfigMgr As ConfigurationManager Set swConfigMgr = swModel.ConfigurationManager swConfigMgr.EnableConfigurationTree = False ' Disable updates ' Modify configurations... swConfigMgr.EnableConfigurationTree = True ' Enable updates again |
7. EnableGraphicsUpdate
This API call stops the SOLIDWORKS graphics window from updating, speeding up operations. Consequently, windows thumbnails may not update when saving files.
Example:
1 2 3 4 5 6 7 8 |
Dim swModelView As ModelView Set swModelView = swModel.ActiveView swModelView.EnableGraphicsUpdate = False ' Disable graphics updates ' Perform intensive operations... swModelView.EnableGraphicsUpdate = True ' Restore updates |
8. UserControlBackground
This API call hides the entire SOLIDWORKS window, maximizing speed.
Example:
1 2 3 4 5 6 7 8 |
swApp.UserControlBackground = True ' Hide SOLIDWORKS window swApp.Visible = False ' Perform operations... swApp.Visible = True swApp.UserControlBackground = False ' Restore visibility |
9. Lock/Unlock Model
This API call locks the user interface while making intensive API calls. API calls like InsertNote may stop working.
Example:
1 |
swModel.Lock ' Lock the model to prevent UI updates<br><br>' Perform complex operations...<br><br>swModel.Unlock ' Unlock the model<br> |
10. Do not use Excel Interop dll
If you’re writing a .NET application (add-in or standalone) and you need to export or import data from Excel, it is better to use a library called EPPlus instead of using the Excel interop dll. You will get a significant performance increase when you make the switch.
By strategically using these API calls, you can significantly improve the performance of SOLIDWORKS macros and add-ins. The key takeaways are:
- Use
CommandInProgress
to minimize unnecessary UI updates. - Leverage
AddToDB
,EnableFeatureTree
, andEnableGraphicsUpdate
for performance gains. - Disable UI updates and background processes where possible (e.g.,
UserControlBackground
,EnableGraphicsUpdate
). - Avoid overusing
DoEvents
, as it can slow down execution.